OAuth2 Authentication
The OAuth2 Authentication enables applications to obtain limited access to a service without requiring the username and password of the actual user of that service. That is, instead of accessing the service directly, OAuth2 introduces an authorization server that is first accessed to obtain a set of temporary and/or limited credentials that can be used to then access the actual resource. These credentials are referred to as access tokens.
OAuth2 support several different mechanisms to obtain these tokens and these mechanisms are referred to as grants. Currently, the following grants are supported:
Client Credentials Grant – This is the recommended flow to use between SL1 and the monitored entity since it is for machine to machine authorization.
Resource Owner Password Credentials Grant – In this case a user name and password is leveraged to obtain the token. NOTE that in this case the username and password are stored within the SL1 system.
The following grant types are not supported:
Authorization Code Grant – This is grant type requires the interaction of a user and thus is not applicable to SL1.
Implicit Grant – This grant is no longer recommended to be used and thus is not supported in the REST Toolkit.
All OAuth2 requests have some basic requirements when making the token request:
Client ID
This required field provides the ClientID (similar to username) when requesting a token. This field is required.
Client Secret
This required field provides the secret (similar to password) when requesting a token. This field is required.
Access Token URL
This required field provides the URL for generating the token that is used in the data request. This field is required.
Request Header
This optional field describes the name of the header to be sent. Default:
Authorization
. This field is required.Token Format
This optional field describes how the content should be sent. The format allows for a single substitution for the token. This substitution occurs when specifying
{}
in the format. For example, if you wanted to useBearer <token>
you would inputBearer {}
. Default:{}
. This field is required.Response Token Key
This required field states the key where the token exists in the returned JSON dictionary. This field is required.
Client auth method
This required field states how the Client ID / Client Secret is sent to the token server. Basic Auth utilizes the Authorization header while Post Body adds this information into the body of the request. This field is required.
Authentication Failure Retry Time
This optional field states the number of seconds the authenticator must wait before retrying the authentication upon failure. Default:
60
. This field is required.Token Refresh Implementation
This field allows the selection of the token refreshing strategy. There are two options
Static
andDynamic
. Default:Dynamic
. This field is required.
Dynamic
allows the user to specify the fieldExpiry Time Key
. TheExpiry Time Key
field is used when retrieving the time-to-live from the authentication request’s response. The next authentication request will occur when the expires time is within two collection polling cycles. For example, if a Dynamic Application has a polling frequency of 5 minutes. Then a new token will be fetched when the saved token’s expiration time is less than 10 minutes.
Static
allows the user to specify a recurring time to refresh their token (in seconds).
Client Credentials
The Client Credentials Grant Type allows the generation of a token for a given set of scopes. See RFC 6749#section-4.4.
To use as your OAuth2 workflow, you must select
Client Credentials
as the value for the Oauth2 Grant Type
dropdown.
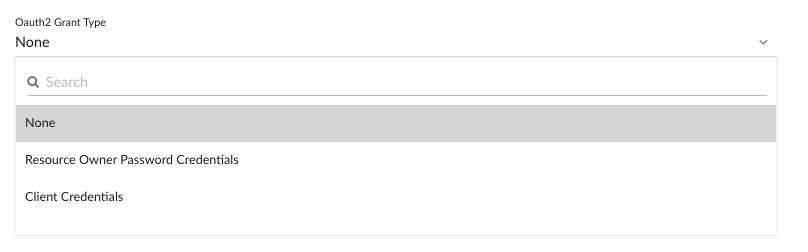
The field included Client Credentials:
Token Scopes
This field supplies all scopes that are required to make your data call. If you require multiple scopes, refer to your token server on how to supply this information. This field is optional.
Example
You need to make a request to a web server that requires a
token from OAuth2 / Client Credentials. The web server expects
the header Auth: Bearer <token>
. The token server requires
the client credentials within the body of the request. The token server
generates the tokens at https://token.info
with client id
of my_client_id, client secret of my_client_secret, owner credential
of OwnerUser / OwnerPass, token scopes “Scope3, Scope4”, and
returns the following format:
{
"token": "<the_token_to_use>",
"expires": "900s"
}
To configure Oauth2 / Client Credential, the following fields would need to be configured:
Client ID:
my_client_id
Client Secret:
my_client_secret
Access Token URL:
https://token.info
Request Header:
Auth
Token Format:
Bearer {}
Response Token Key:
token
Client auth method:
Post Body
Token Scopes:
Scope3, Scope4
Note
Given the token response format above, the token refresh
implementation could be set to Dynamic
. The Expiry
Time Key
would be expires
in this example. Alternatively,
a Static
time of 900
could be set manually.
Example - AWS Cognito
The following example shows a credential that is taken from a working SL1 system that is used to communicate with an AWS Lamda function behind an AWS API Gateway and uses the AWS Cognito service for OAUTH2 authentication.
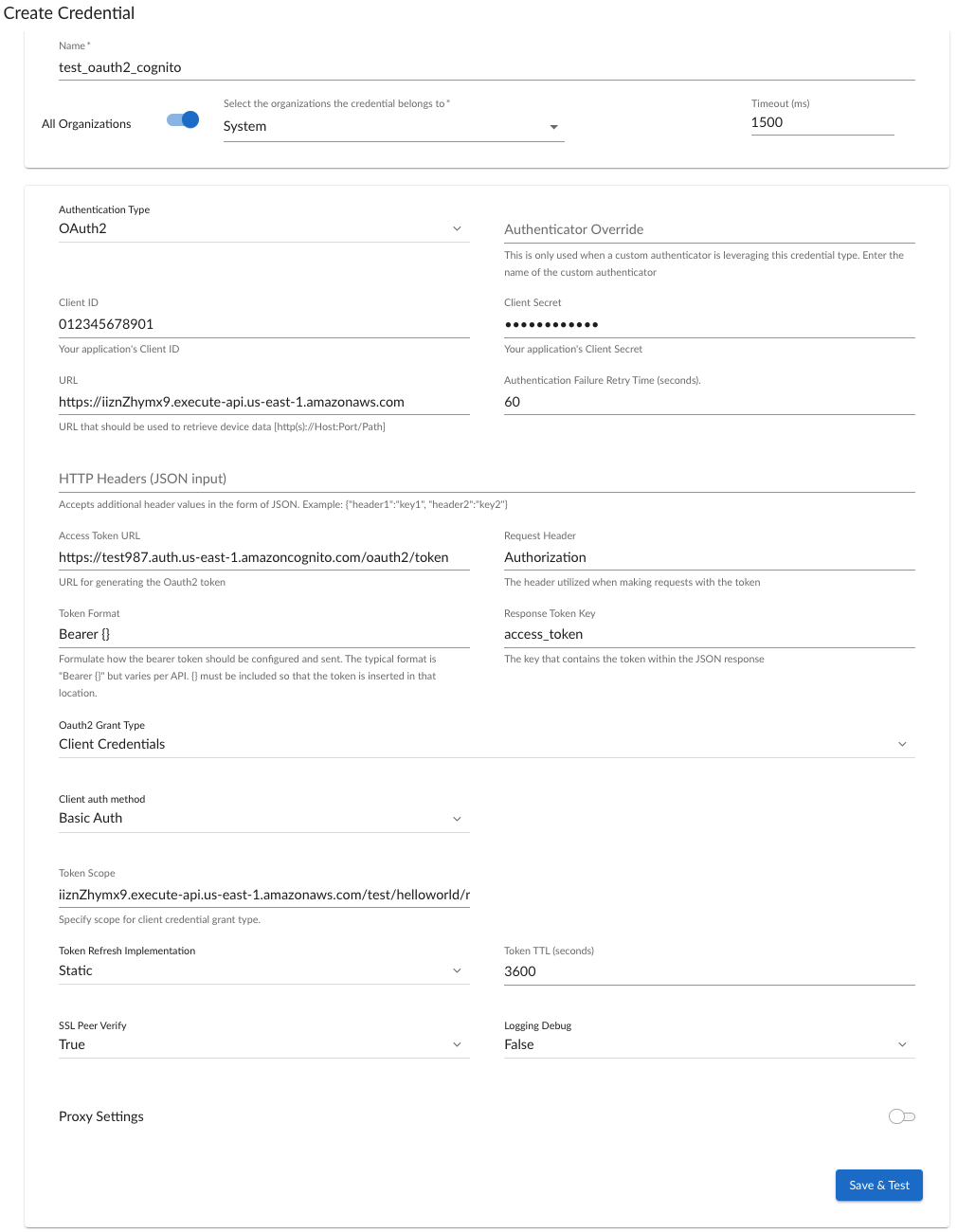
Authentication Type
In the above example, Oauth2 is selected from the drop down list.
The next step is to enter the Client ID
and the Client Secret
.
In this example this was obtained from the Cognito Service (Specifically
from the App client set up for this example that is associated with a user pool).
URL
The next field is the URL
of the API that will be queried by SL1 to obtain the
data for the various collection objects. In this example, the URL
points to the API
gateway. Given the following snippet argument for an SL1 collection object with
the above credential:
steps:
- http:
uri: "/test/helloworld"
SL1 will query
https://iizn2hymx9.execute-api.us-east-1.amazonaws.com/test/helloworld
Access Token URL - This is the URL of the authentication server. SL1 queries this URL to obtain the access token. In the above example, this information comes from the Cognito User Pool App Integration Cognito domain.
Request Header -
This fields defines the header used to send the token. This is normally
Authorization
. That is when SL1 sends a request to the API it will include an
Authorization header which has the token.
Token Format -
The Token Format allows to specify what this is but normally should be Bearer {}
.
The response token key identifies the key that contains the token in the response
from the authentication server. This is normally access token
.
Oauth2 Grant Type - For this flow this Client Credentials is selected from the drop down list,
Client Auth Method - Only Client Password authentication method is supported. However, SL1 support two mechanisms for sending the client password to the authentication server as follow:
Basic Authentication -
In this case SL1 takes the Client Id and Client Secret and
leverages HTTP basic authentication scheme. This is generally the preferred approach.
Send the Client Id
and Client Secret
in the body of the request using
Content-Type: application/x-www-form-urlencode
Resource Owner
The Resource Owner Grant Type enables the generation of a token for a given username, password, and scopes. See RFC 6749#section-4.3.
To use this grant type, you must select
Resource Owner Password Credentials
as the value for the
Oauth2 Grant Type
drop-down list.
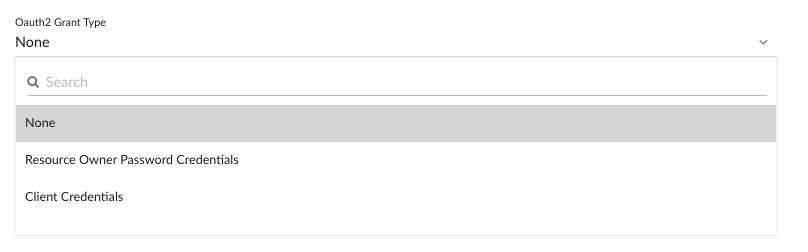
The required fields to utilize Resource Owner include:
Resource Owner Username
This required field states the username that will own the token. This field is required.
Resource Owner Password
This required field states the password for the given username of the token. This field is required.
Token Scopes
This field supplies all scopes that are required to make your data call. If you require multiple scopes, refer to your token server on how to supply this information. This field is optional.
Additional body Parameters
This field allows for any additional parameters to be included with the token request. This value should be a valid JSON dictionary. This field is optional.
Example
You need to make a request to a web server that requires a
token from OAuth2 / Resource Owner. The web server expects
the header Auth: Bearer <token>
. The token server requires
the client credentials as part of basic auth. The token server
generates the tokens at https://token.info
with client id
of my_client_id, client secret of my_client_secret, owner credential
of OwnerUser / OwnerPass, token scopes “Scope3, Scope4”, and
returns the following format:
{
"token": "<the_token_to_use>",
"expires": "900s"
}
To configure Oauth2 / Resource Owner, the following fields would need to be configured:
Client ID:
my_client_id
Client Secret:
my_client_secret
Access Token URL:
https://token.info
Request Header:
Auth
Token Format:
Bearer {}
Response Token Key:
token
Client auth method:
Basic Auth
Resource Owner Username:
OwnerUser
Resource Owner Password:
OwnerPass
Token Scopes:
Scope3, Scope4